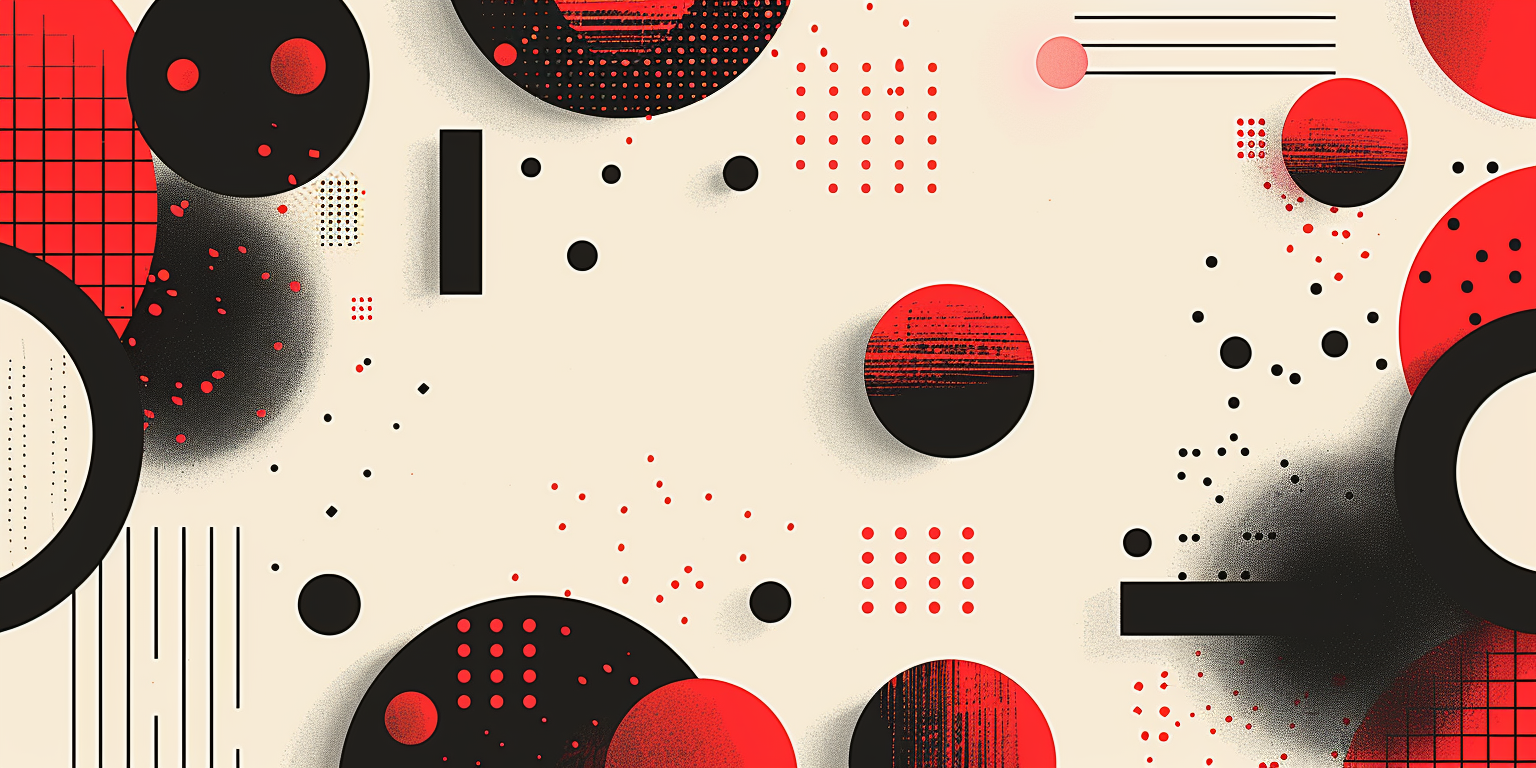
Dgraph Lambda in Dgraph Cloud is a serverless framework that lets you run Javascript or Typescript function in response to GraphQL queries or mutations. This helps in simplifying development - now you can embed business logic or trigger events on GraphQL queries/mutations.
These are the broad classification of use cases that you can solve with Dgraph Lambda out of the box:
Lambda can be used to notify your app users of events. GraphQL operations can be modeled as events. For example, if you are building a user subscription form, you can trigger an email to confirm the subscription when people sign up by creating an event for executing a mutation (creating user object). This can be easily done with lambda by triggering a simple HTTP request to your email delivery service like Twilio Sendgrid. (Checkout sample code).
Steps:
You can use lambda to automate time-consuming jobs, data pipelines, or machine learning models that can be asynchronously executed. For example, if you have a text classifier machine learning model service, you can use lambda to call this service. This will trigger the classification service. On completion of model service execution, the result can be written to the database based on the incoming payload.
Steps:
You can perform simple data derivations & lightweight transformation on lambda. For example - aggregation of fields, string concatenations, rounding off numbers, etc.
Steps:
You can perform data sanitization using lambda. For example - handling missing data, trimming text, filter unwanted values, etc.
Steps:
A backend that allows users to store subscriber data (name & email) and sends a welcome email using SendGrid API to the email being stored.
Schema
type Subscriber {
name: String!
email: String!
}
input CreateSubscriberInput {
name: String!
email: String!
}
type Mutation {
createSubscriber(subscriber: CreateSubscriberInput): Subscriber @lambda
}
Lambda
self.addGraphQLResolvers({
"Mutation.createSubscriber": createSubscriber,
});
async function createSubscriber({ args, graphql }) {
const { subscriber } = args;
const results = await graphql(
`
mutation($name: String!, $email: String!) {
addSubscriber(input: { name: $name, email: $email }) {
subscriber {
email
name
}
}
}
`,
{ ...subscriber }
);
const { email, name } = subscriber;
sendMail(email, name);
return results.data.addSubscriber.subscriber[0];
}
function sendMail(email, name) {
var myHeaders = new Headers();
myHeaders.append(
"Authorization",
"Bearer {{YOUR_TOKEN_FROM_SENDGRID}}"
);
myHeaders.append("Content-Type", "application/json");
var raw = JSON.stringify({
personalizations: [
{
to: [
{
email: email,
name: name,
},
],
subject: "Welcome",
},
],
content: [
{
type: "text/plain",
value: "You are added to the subscription!",
},
],
from: {
email: "[email protected]",
name: "Thiyagaraj T",
},
});
var requestOptions = {
method: "POST",
headers: myHeaders,
body: raw,
redirect: "follow",
};
fetch("https://api.sendgrid.com/v3/mail/send", requestOptions)
.then((response) => response.text())
.then((result) => console.debug(result))
.catch((error) => console.error("error", error));
}
The example creates a backend that stores subscribers’ data - The GraphQL schema exposes a custom mutation createSubscriber
that is annotated with @lambda
directive.
The lambda script registers a javascript function against createSubscriber
mutation. This function will be invokend whenever a createSubscriber
mutation is executed.
Dgraph Lambda allows you to simplify development and build a reactive backend. You can trigger webhooks to connect with your other microservices, queues, third-party services and do more than database operations. Try Dgraph Lambda on Dgraph Cloud now!
https://dgraph.io/blog/post/lambda-slash-graphql/
https://dgraph.io/docs/graphql/lambda/overview/